Setup Liveness SDK
Getting Started
Prerequisites
Please install the following:
- Node version
16.x
- Yarn as a package manager
- This library is built with
React v16
, so make sure your project uses the compatible version of React.
Before installing this package please add the.npmrc
file in your root directory with the following value
PS: GITHUB_REGISTRY_TOKEN will be provided by AppMan
@appman-agm:registry=https://npm.pkg.github.com/
//npm.pkg.github.com/:_authToken=${GITHUB_REGISTRY_TOKEN}
Installation
To install the Liveness SDK, ensure you have a React project set up. Then, use the following command:
npm install @appman-agm/mac-liveness
Configuration
To utilize the features of the library, you need to configure it by calling the create() function. This function initializes a liveness SDK instance with the specified configuration.
In the configuration, you provide the following parameters:
kycBaseUrl
: The base URL for KYC (Know Your Customer) service.kycPublicKey
: The public key for KYC service.imagePath
: The path to the folder containing images. (optional)translations
: Object containing translations for multiple languages. (optional)lang
: Language setting. (optional)theme
: Theme customization, including primary colour. (optional)getCustomization
: Function for advanced customization options. (optional)- Here's an example of how to create the configuration:
// App.tsx
import { create, type LivnessCutomization } from '@appman-agm/mac-liveness'
import type { LivenessInstance, livenessCustomization } from '@appman-agm/mac-liveness'
import en from './locales/en.json'
import th from './locales/th.json'
const instanceConfig: LivenessInstance = await create({
kycBaseUrl: KYC_BASE_URL,
kycPublicKey: KYC_PUBLIC_KEY,
imagePath: '/images',
lang: 'en',
translations: { en, th },
theme: { primary: '#2f4E4E'},
getCustomization: (customization: LivenessCustomization) => {
// Additional customization logic can be implemented here
return customization
}
})
Replace placeholders such as KYC_BASE_URL and KYC_PUBLIC_KEY with actual values relevant to your configuration. This sets up the necessary parameters for starting liveness sessions.
Initialization
After creating the configuration, call the initialize() function to set up the SDK:
instanceConfig.initialize()
Starting Liveness Verification
To begin the liveness verification process, you can choose between starting a standard liveness session
or a 3D face comparison session
.
Starting a Liveness Session
instanceConfig.startLivenessSession(verificationId)
Starting a 3D Face Comparison Session
instanceConfig.start3DCompareSession(verificationId)
Customization Guide
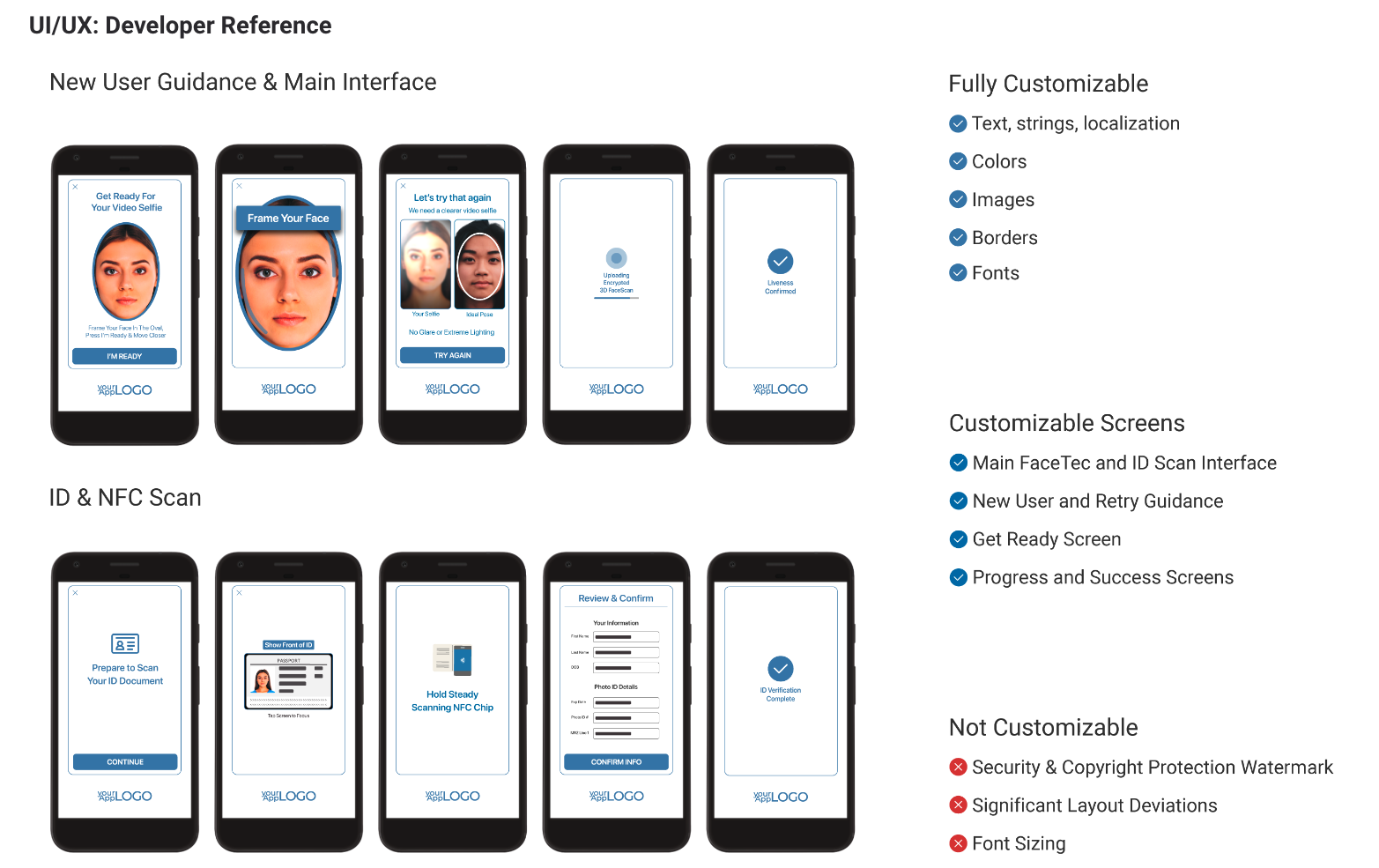
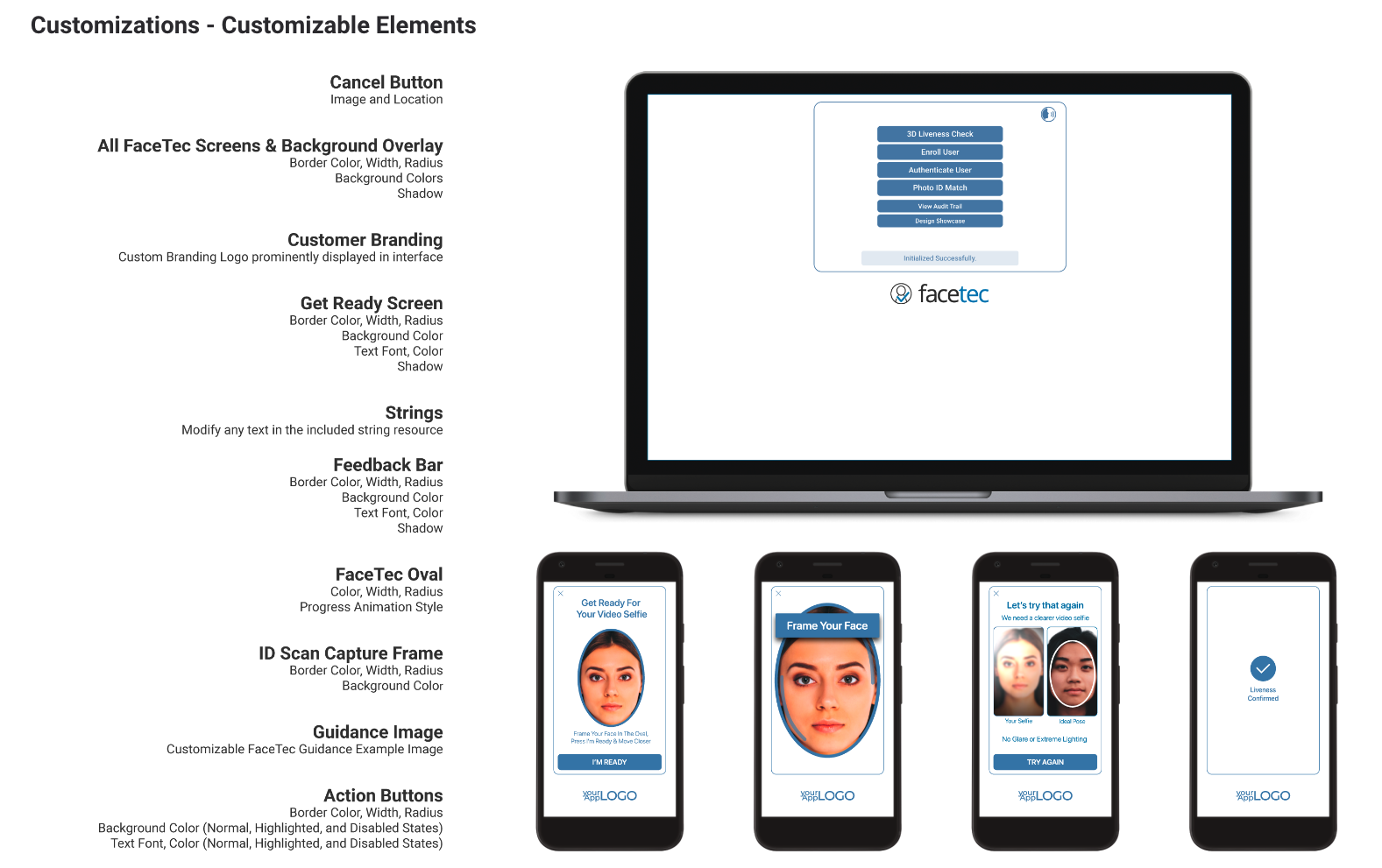
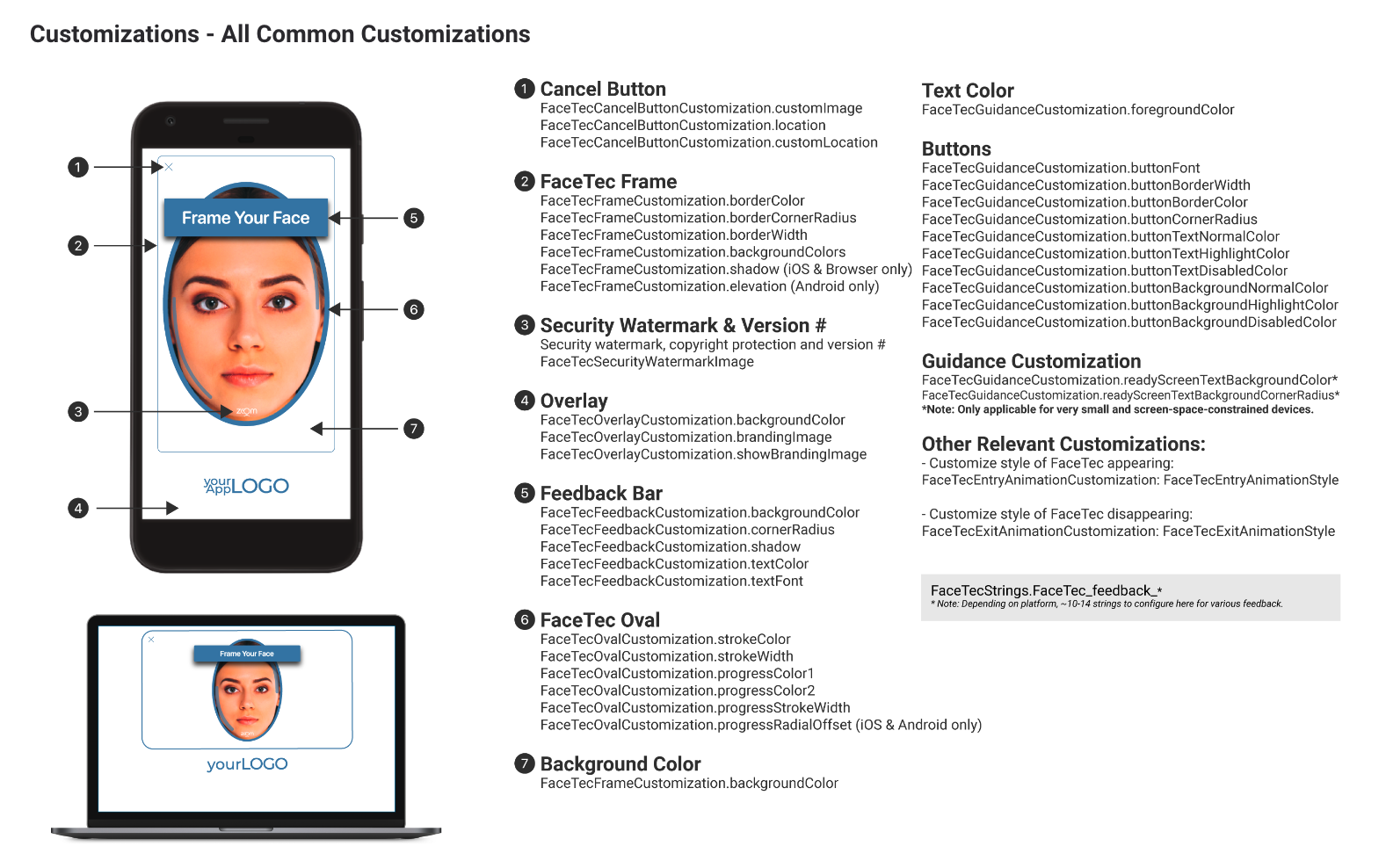
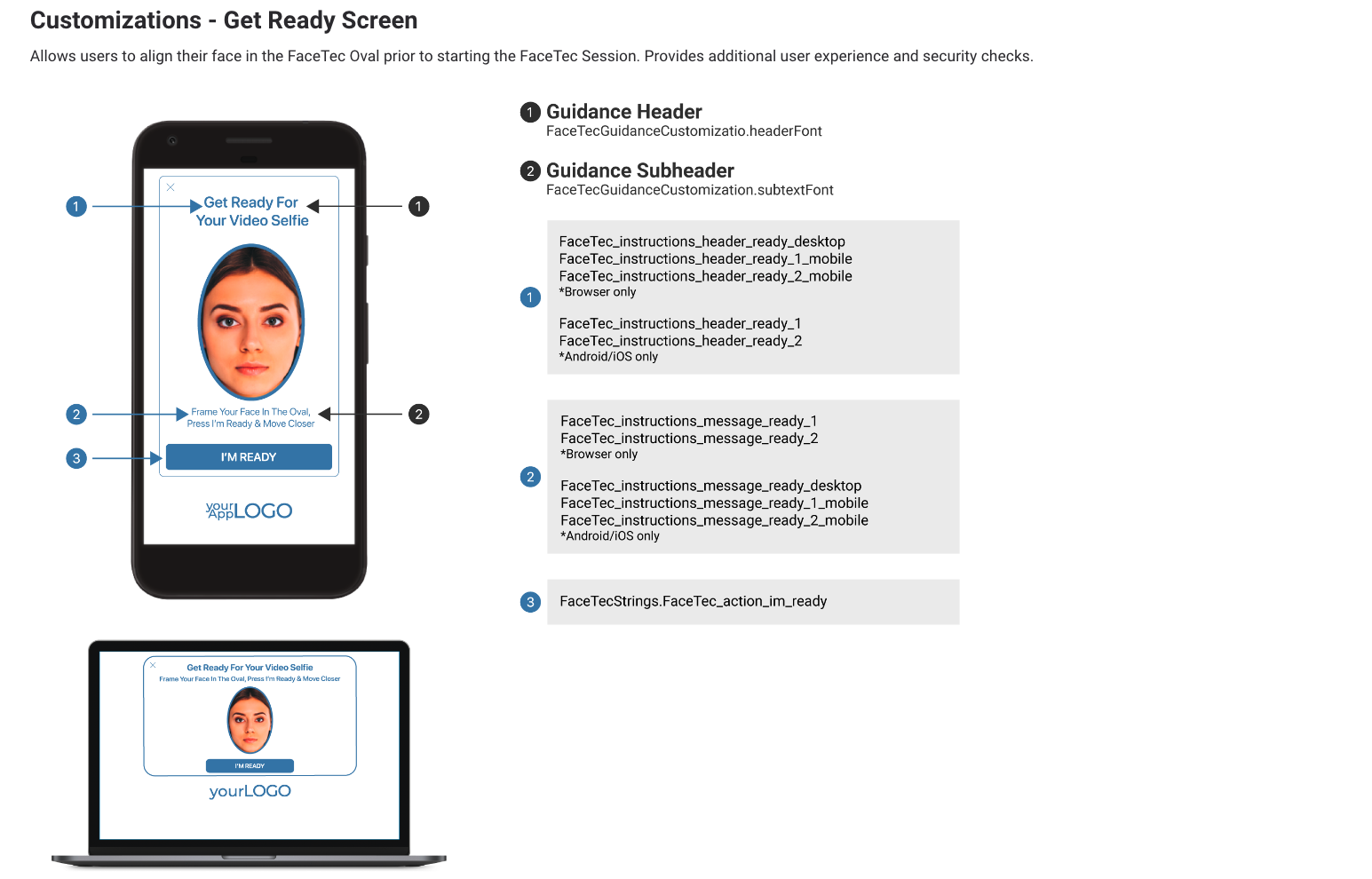
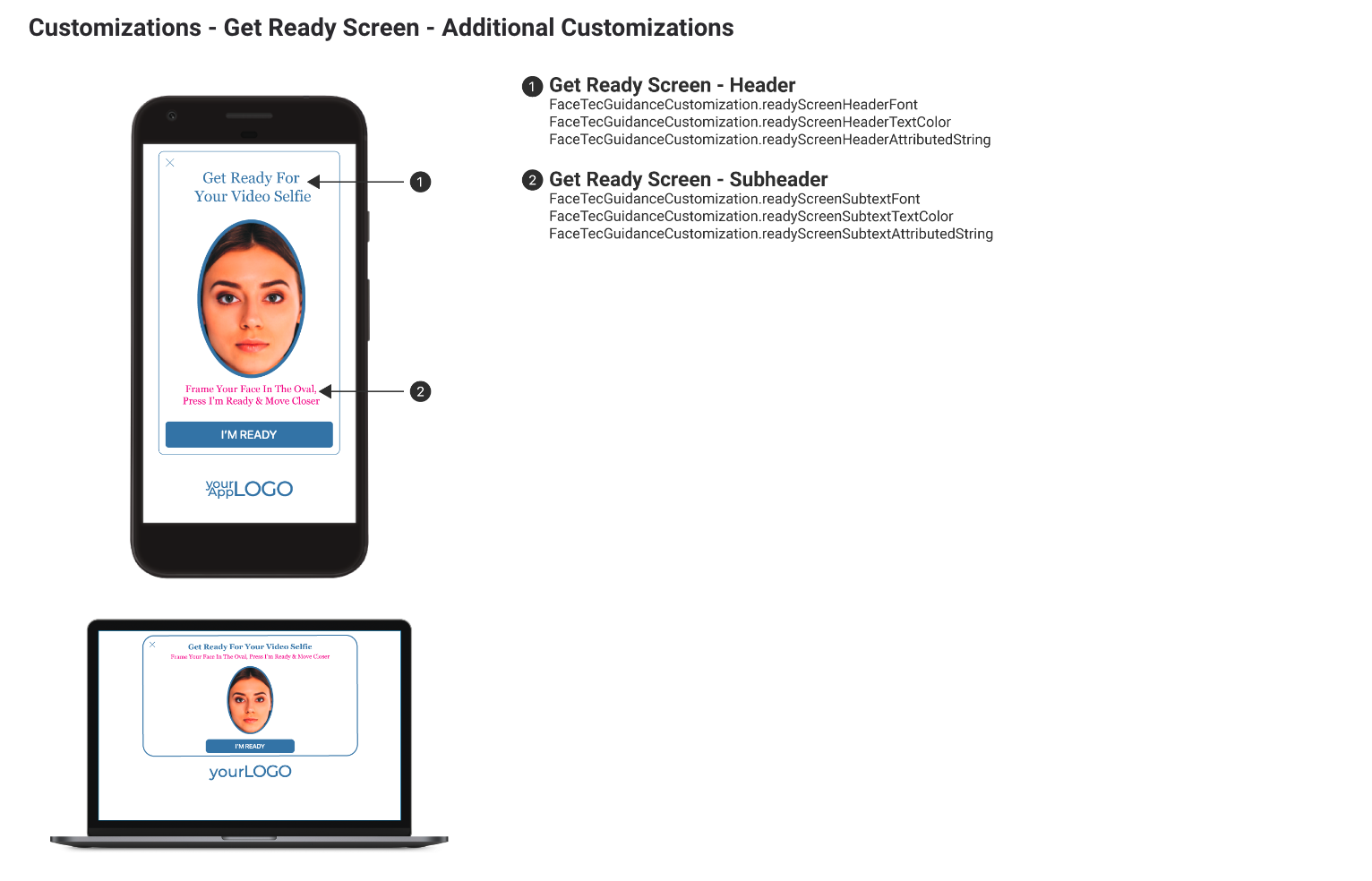
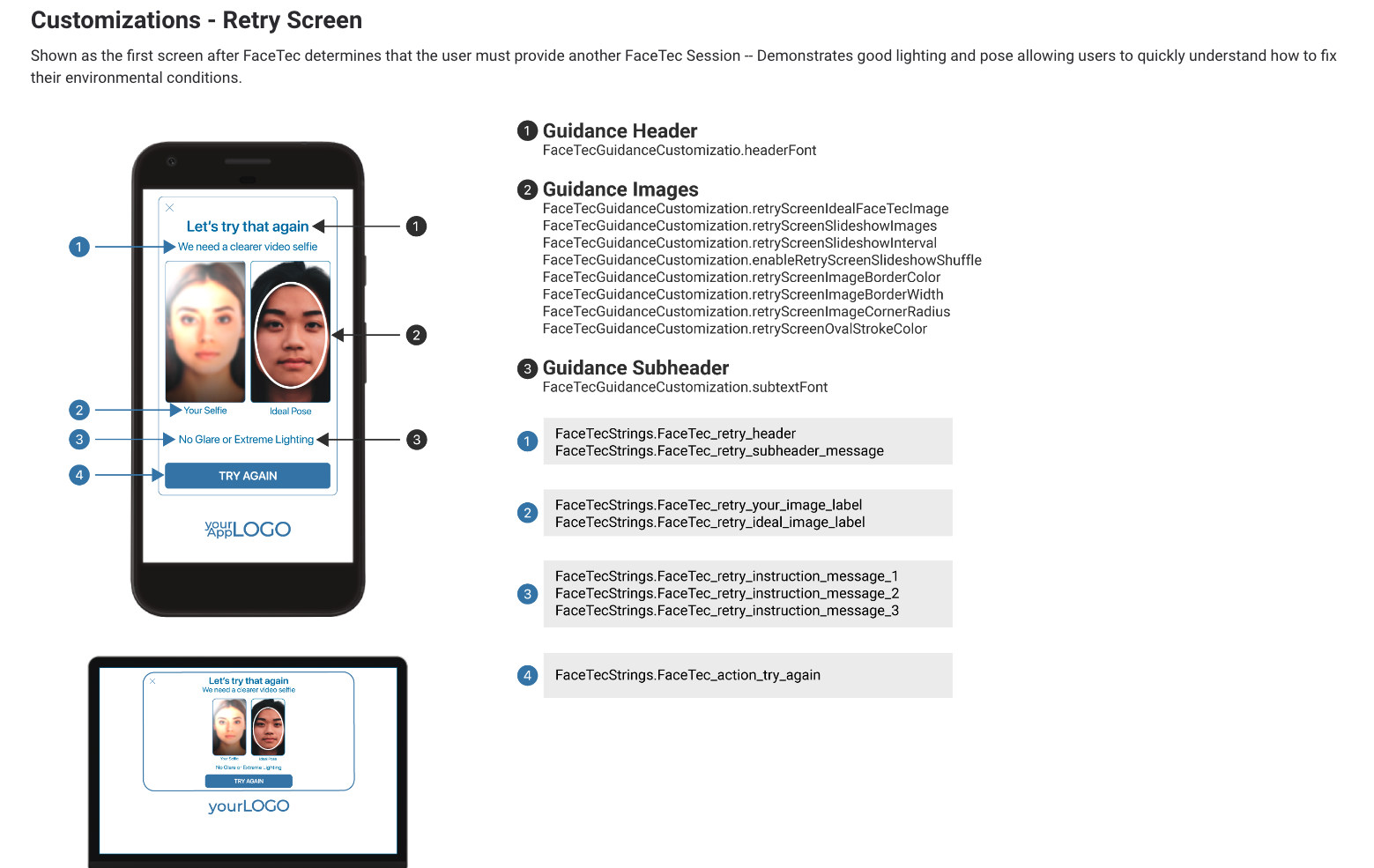
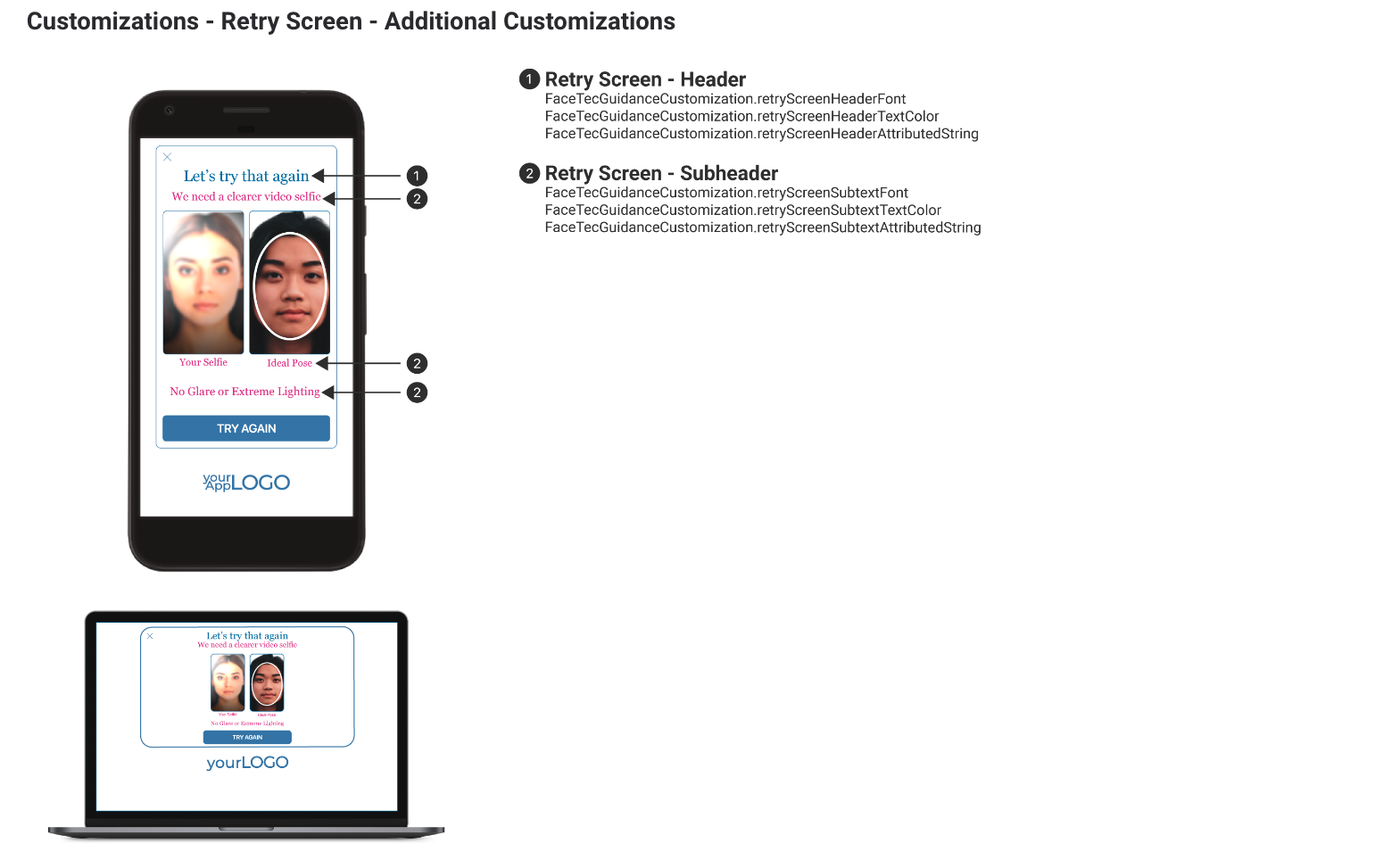
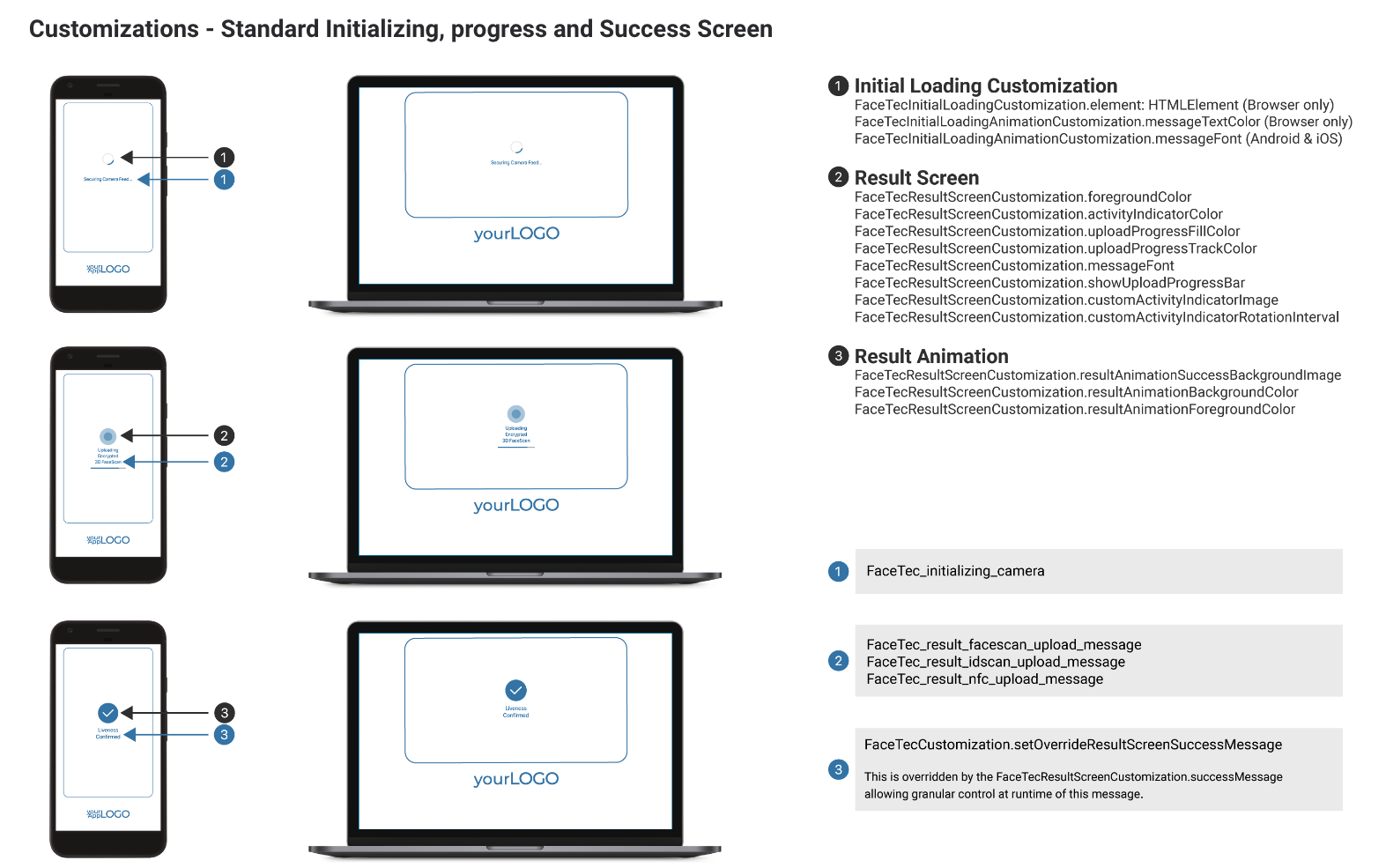
Updated over 1 year ago